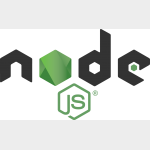

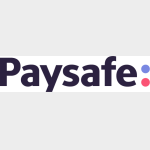
Integrate NodeJS and Paysafe
Trexle connects NodeJS to Paysafe and 100+ other payment gateways using a single plugin for one time annual fee
Paysafe and NodeJS Integration Guide
Paysafe integration credentials are API key, Account ID and Merchant Security Key.
To get your Paysafe credentials Sign in to your Paysafe merchant account then go to Settings > API Key and copy the fields under Username and Password.
Now go to the Accounts tab and copy the account number.
An actual Paysafe Merchant Security Key is taken from your Paysafe introductory email message.
Take the credentials you just obtained from Paysafe and navigate to Trexle dashboard, click Payments Gateways and select Paysafe from the drop down menu as shown below.
Fill into your Paysafe credential and click Add Gateway. Click the Activate button next to Paysafe, and it will give you a success message and the button will turn into green. You should see something like below.
In your NodeJS project, open a terminal and issue the following command:
npm install trexlejs
If you want to test, consider writing the following example in a test.js file:
var Trexle = require('trexlejs');
var trexle = Trexle.setup({
key: ‘your-api-key’,
production: false
});
trexle.createCharge({
amount: 400,
currency: ‘usd’,
description: ‘test charge’,
email: ‘[email protected]’,
ip_address: ‘66.249.79.118’,
card: {
number: ‘4242424242424242’,
expiry_month: 8,
expiry_year: 2018,
cvc: 123,
name: ‘John Milwood’,
address_line1: ‘423 Shoreline Park’,
address_city: ‘Mountain View’,
address_postcode: 94043,
address_state: ‘CA’,
address_country: ‘US’
}
}, function (response) {
console.log(response.body);
});
Then run the code:
node test.js
When using the test card numbers, you can use any date in the future for the expiry date (e.g., 11/27).
You can use any 3 (or 4 for Amex cards) digit number for the CVV in the merchant test environment.
Visa
- 4530910000012345 3D Secure: X Issuing Country:CA
- 4510150000000321 3D Secure:X Issuing Country:CA
- 4500030000000004 3D Secure:YES Issuing Country:CA
- 4003440000000007 3D Secure:YES Issuing Country:CA
- 4515031000000005 3D Secure:YES Issuing Country:CA
- 4538261230000003 3D Secure:YES Issuing Country:CA
- 4037112233000001 3D Secure:X Issuing Country:US
- 4037111111000000 3D Secure:YES Issuing Country:US
- 4107857757053670 3D Secure:YES Issuing Country:UK
Visa Debit
- 4724090000000008 3D Secure:X Issuing Country:CA
- 4835641100110000 3D Secure:YES Issuing Country:CA
- 4900880000000008 3D Secure:YES Issuing Country:US
- 4900770000000001 3D Secure:X Issuing Country:US
- 4206720389883775 3D Secure:YES Issuing Country:UK
Visa (Electron)
- 4917480000000008 3D Secure:X Issuing Country:UK
- 4917484589897107 3D Secure:X Issuing Country:UK
Mastercard
- 5191330000004415 3D Secure:X Issuing Country:CA
- 5457490000008763 3D Secure:X Issuing Country:CA
- 5411420000000002 3D Secure:YES Issuing Country:CA
- 5258110000000005 3D Secure:YES Issuing Country:CA
- 5192810000000009 3D Secure:YES Issuing Country:CA
- 5100400000000000 3D Secure:X Issuing Country:US
- 5200400000000009 3D Secure:YES Issuing Country:US
- 5186750368967720 3D Secure:YES Issuing Country:UK
Mastercard Debit (Maestro)
- 6277411477100000 3D Secure:YES Issuing Country:CA
- 6277411477200002 3D Secure:X Issuing Country:CA
- 5036150000001115 3D Secure:X Issuing Country:US
- 5036160000001114 3D Secure:YES Issuing Country:US
- 5573560100022200 3D Secure:YES Issuing Country:UK
- 6759950000000162 3D Secure:X Issuing Country:UK
American Express
- 373511000000005 3D Secure:X Issuing Country:CA
- 373522010100107 3D Secure:YES Issuing Country:CA
- 370123456789017 3D Secure:X Issuing Country:US
- 370123456789116 3D Secure:YES Issuing Country:US
- 375529360131002 3D Secure:X Issuing Country:Ireland
Discover
- 6011234567890123 3D Secure:X Issuing Country:US
JCB
- 3569990000000009 3D Secure:X Issuing Country:Japan
Paysafe Supported Countries


Paysafe Supported Payment Processing Actions
✔ Authorize
✔ Capture
✔ Void
✔ Credit
✔ Recurring
✔ Card Store
Key Features
About Trexle
Trexle is a powerful online recurring subscription billing platform that integrate Paysafe and other +100 payment gateways with NodeJS and other dozen of e-commerce platforms.
About Paysafe
Paysafe, formerly known as Optimal Payments Netbanx, offers a full suite of credit and debit card functionality to meet all online merchants’ card-processing needs. With a single integration they can process a complete range of card requests, including authorizations/purchases, settlements, refunds, card verifications, standalone credits, and more. All card payment requests are protected by Paysafe’s airtight risk- and fraud-management services.
About NodeJS
Node.js is an open-source, cross-platform JavaScript runtime environment for executing JavaScript code server-side, and uses the Chrome V8 JavaScript engine. Historically, JavaScript was used primarily for client-side scripting, in which scripts written in JavaScript are embedded in a webpage's HTML, to be run client-side by a JavaScript engine in the user's web browser. Node.js enables JavaScript to be used for server-side scripting, and runs scripts server-side to produce dynamic web page content before the page is sent to the user's web browser.